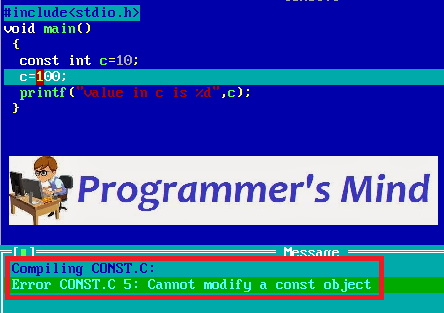
What is a Constant??
Constant is an identifier whose value cannot be changed during program execution.
Previous Topic:
How to use Constants in Programming??There are two ways to use constants in C Programming.They are
1.Using const Keyword
2.using #define (macros)
Program for defining constant using 'const':
#include<stdio.h>
void main()
{
const int c=10;
printf("value in c is %d",c);
}
If you try to modify the value of c in above program then compiler will generate an error as follows
How to change the value of a constant??
We can change the value of a constant using pointers as shown in below program
#include<stdio.h>
void main()
{
const int c=10;
int *ptr;
ptr=&c;
*ptr=100;
printf("value in c is %d",c);
}
Output of this program is

Syntax for using #define:
#define CONSTANT VALUEProgram for defining constant using '#define':
#include<stdio.h>
#define k 2
void main()
{
int s;
s=k*k+k;
printf("value of s is %d",s);
}
Output of the above program is "Value of s is 6"
If you define a constant using #define then that constant is replaced by the corresponding value before compilation as #define is a preprocessing directive.
0 comments:
Post a Comment