Next topic after control statements is arrays. Before going to learn syntax and usage of arrays first we have to know the
Definition of an Array:
An Array is a collection of homogeneous data elements.Description about Arrays in C:
Array in C programming is a data structure to store the elements of same datatype in a sequential order.Size of an array should be specified at the time of declaration since arrays in c programming are static. Elements of an array are stored in a continuous memory locations. We can also access the element of our interest by using index of that element in array. Indices are the integer numbers given to each array element in a sequential order. An indices of array starts from 0 i.e., first array element will have the index 0,second array element will have 1 and so on.
Syntax for declaring an Array in C
datatype arrayname [ arraysize ];
Example:
int a[10];
Initializing of Arrays in C programming
int a[]={1,2,3,4,5};
or
int a[5]={1,2,3,4,5};
If you initialize an array in the above manner then the elements will be stored as follows
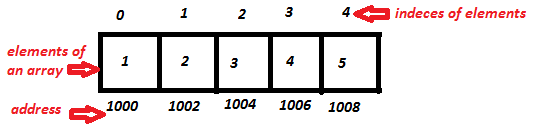
For reading of an array we need a loop in order to access the elements with their indices. Program for reading and printing an array in C programming uses for loop.
Program for reading and printing an array in C
#include<stdio.h>
void main()
{
int a[5],i;
for(i=0;i<5;i++)
{
printf("Enter a[%d]:\n",i);
scanf("%d",&a[i]);
}
printf("ELEMENTS OF ARRAY");
for(i=0;i<5;i++)
{
printf("\na[%d] is %d",i,a[i]);
}
}
Output for the above program:
.png)
What happens when we are trying to access array beyond its size??
Visit:Hidden facts about arrays
In above all examples only integer arrays are mentioned. We can also use arrays of other datatypes such as float,double But character arrays are special arrays known as strings in C programming.
We can also store arrays as elements of another array i.e., array of arrays. This concept is nothing but multi-dimensional arrays.
0 comments:
Post a Comment