In C, Control statements are of two types
If the condition of the 'if' is true then the control of the program will enter into the block of statements otherwise the block will be skipped.The condition will return either 0 or 1.If the condition is true then the 1 will be returned and if it is false then 0 will be returned.As Boolean datatype is not available in C. '0' is treated as false and any value other than '0' (it can be negative also) is treated as true.
- Conditional Statements
- Unconditional Statements
Conditional Statements are again of two types
- Sequential
- Iterative
Sequential Control Statements are simple if,if-else,nested if,else if ladder and switch
Simple if:
Simple If is a sequential conditional control statement with following syntax
if(Condition)
{
statement-1;
statement-2;
.
.
.
statement-n;
}

Example Program
#include<stdio.h>
void main()
{
int a=10;
if(a==10)
{
printf("a value is 10");
}
}
Output of above program is a value is 10
as the condition a==10 is true in above program.
if-else:
Syntax for if-else statement
if(condition)
{
Block of statements;
}
else
{
Block of statements;
}

if-else also checks for the condition same as in Simple If. If the condition is true then the block of statements in if block will be executed otherwise the block of statements in the else block will be executed.
Example Program (Even Number Program)
#include<stdio.h>
void main()
{
int n;
printf("Enter Number:\n");
scanf("%d",&n);
if(n%2==0)
{
printf("%d is an even Number",n);
}
else
{
printf("%d is an Odd Number",n);
}
}
void main()
{
int n;
printf("Enter Number:\n");
scanf("%d",&n);
if(n%2==0)
{
printf("%d is an even Number",n);
}
else
{
printf("%d is an Odd Number",n);
}
}
Interested Program Using if else
Nested if:
If an if statement is placed in another if statement then that is known as nested if statements
Syntax:
if(Condition-1)
{
if(Condition-2)
{
statements;
statements;
}
else
{
statements;
statements;
}
}
else
{
if(Condition-3)
Here first if condition is checked if it is true then it enters in to the block and checks for the condition if there is another if inside if block.
Example Program: Biggest of three numbers
#include<stdio.h>
void main()
{
int a,b,c;
printf("Enter three Numbers:\n");
scanf("%d %d %d ",&a,&b,&c);
if(a>b)
{
if(a>c)
{
printf("%d is Biggest",a);
}
else
{
printf("%d is Biggest",c);
}
}
else
{
if(b>c)
{
printf("%d is Biggest",b);
}
else
{
printf("%d is Biggest",c);
}
}
}
else
{
if(Condition-3)
{
statements;
statements;
}
else
{
statements;
statements;
}
}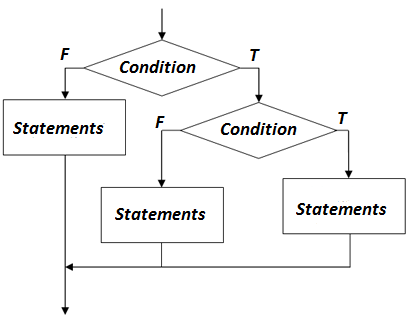
Here first if condition is checked if it is true then it enters in to the block and checks for the condition if there is another if inside if block.
Example Program: Biggest of three numbers
#include<stdio.h>
void main()
{
int a,b,c;
printf("Enter three Numbers:\n");
scanf("%d %d %d ",&a,&b,&c);
if(a>b)
{
if(a>c)
{
printf("%d is Biggest",a);
}
else
{
printf("%d is Biggest",c);
}
}
else
{
if(b>c)
{
printf("%d is Biggest",b);
}
else
{
printf("%d is Biggest",c);
}
}
}
else if Ladder:
Syntax:
if(condition-1)
{
}
else if(condition-2)
{
}
.
.
.
.
.
else if(condition-n)
{
}
else
{
}

In else if ladder first if condition is checked if it is true then the block of statements are executed and then program control transfers to the next statements after else if ladder.If the condition is false then the next if condition is checked(else if).If all the conditions are false then final else block of statements will be executed.
Example Program:
void main()
{
int n;
scanf("%d",&n);
if(n==0)
{
printf("value of n is 0");
}
else if(n>0)
{
printf("value of n is greater than 0");
}
else
{
printf("value of n is less than 0");
}
}
Try to write a program to execute statements in Both if and else Blocks
Previous Topic:
Constants in C
Operators In C
Next Topic:
Switch Statement in C
Try to write a program to execute statements in Both if and else Blocks
Previous Topic:
Constants in C
Operators In C
Next Topic:
Switch Statement in C
0 comments:
Post a Comment